springcloud从入门到放弃
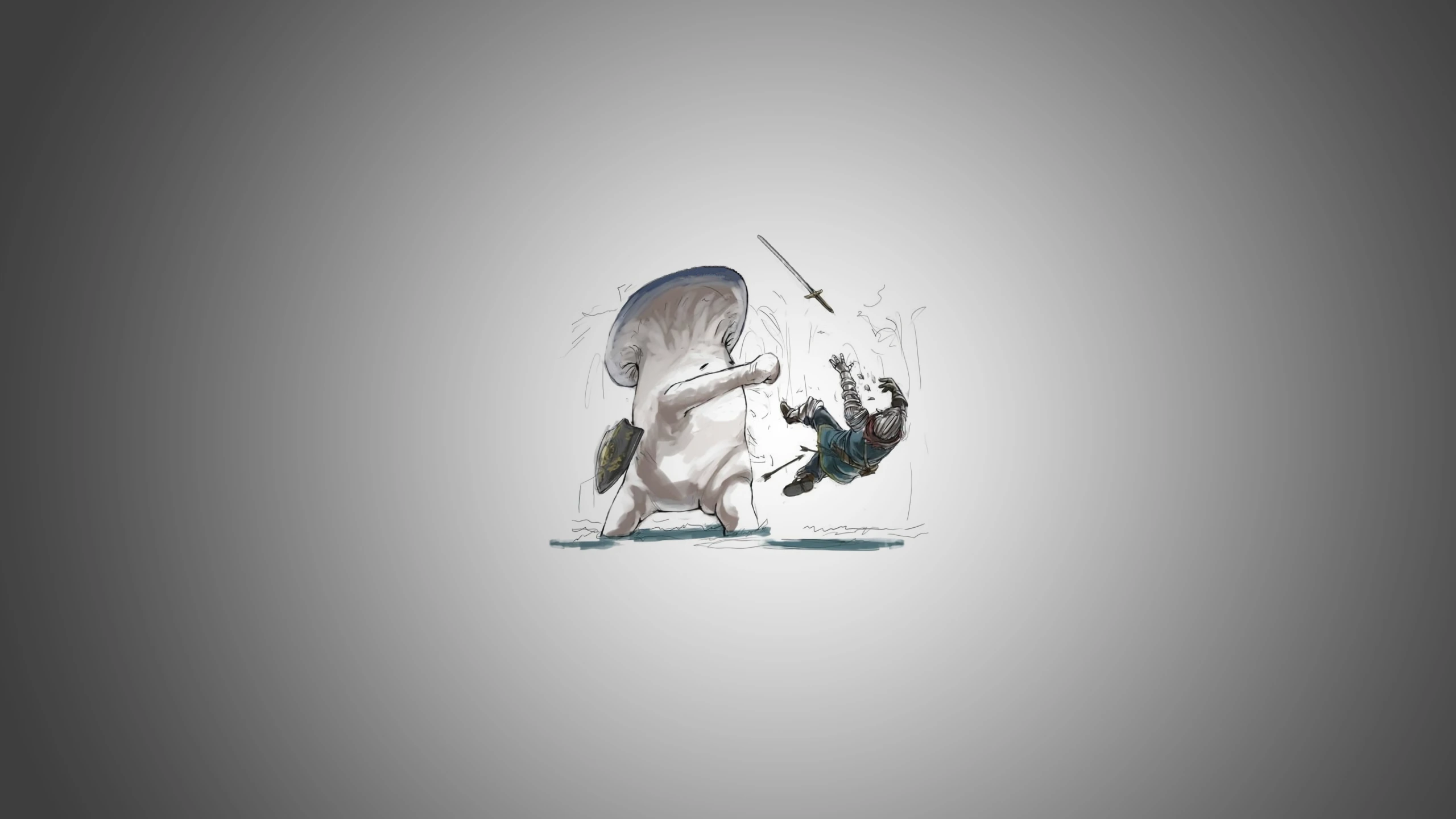
springcloud从入门到放弃
caolibinspringcloud从入门到放弃
gateway
网关的核心功能特性:
- 请求路由
- 权限控制
- 限流
网关就像是看门大爷,只有校验身份的请求才能访问到后面的微服务
Spring Cloud Gateway
是一个基于 Spring 5、Spring Boot 2 和 Project Reactor 的 API 网关,以下是使用 Spring Cloud Gateway 的基本步骤:
- 添加依赖:在 Maven 的
pom.xml
文件中,添加 Spring Cloud Gateway 的依赖。
|
- 配置路由:在
application.yml
或application.properties
文件中,配置路由规则。例如,将所有以/reader/
开头的请求转发到reader-service
服务。
|
- 添加JWT拦截器
|
启动 Gateway:运行 Spring Boot 应用,启动 Gateway。
发送请求:发送请求到 Gateway,Gateway 会根据配置的路由规则,将请求转发到对应的服务。
以上就是使用 Spring Cloud Gateway 的基本步骤。在实际使用中,可能还需要配置其他的功能,例如过滤器、限流、熔断等。
openfeign
基本使用
引入依赖: 在
pom.xml
文件中添加以下依赖:<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<version>2.2.5.RELEASE</version>
</dependency>创建 Feign 客户端接口: 在使用 OpenFeign 时,我们需要创建一个 Feign 客户端接口,用于定义我们想要调用的服务接口。例如:
@FeignClient(name = "user-service")
public interface UserServiceClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") Long id);
@PostMapping("/users")
User createUser(@RequestBody User user);
}注入 Feign 客户端: 在你的控制器中,使用
@Autowired
将UserServiceClient
注入,并通过该客户端接口调用远程服务。例如:@RestController
public class UserController {
@Autowired
private UserServiceClient userServiceClient;
@GetMapping("/users/{id}")
public User getUserById(@PathVariable("id") Long id) {
return userServiceClient.getUserById(id);
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userServiceClient.createUser(user);
}
}启用 Feign: 在 Spring Boot 应用程序的启动类上添加
@EnableFeignClients
注解:@SpringBootApplication
@EnableFeignClients
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
通过使用 OpenFeign,我们可以更加便捷地编写 HTTP 服务客户端,简化了开发流程。
配置连接池
添加依赖: 首先,在
pom.xml
文件中添加Apache HttpClient
的依赖,以替换 OpenFeign 默认的底层客户端 HttpURLConnection:<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>配置连接池属性: 在
application.properties
或application.yml
中配置连接池的相关属性。你可以根据需求设置以下属性:feign.httpclient.connection-manager.max-total=20
启用 HttpClient: 在 Spring Boot 应用程序的启动类上添加
@EnableFeignClients
注解:@SpringBootApplication
@EnableFeignClients
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}